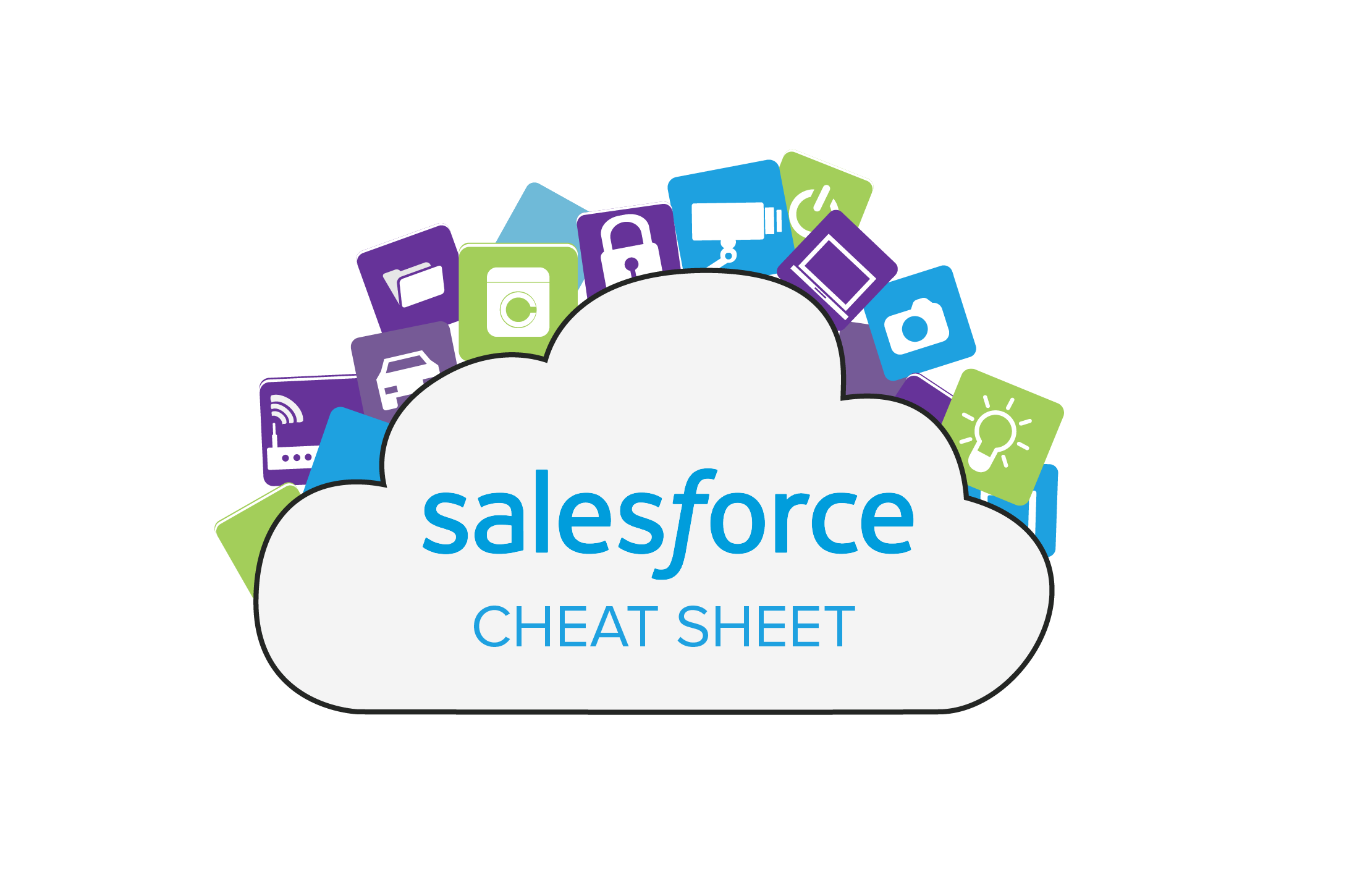
Build secure Salesforce web applications and pass the SFDC AppExchange security review.
As with any web application, it’s important to follow secure development practices when building an application on the Salesforce platform. This is especially important if you plan to release your application on the AppExchange, which requires a mandatory security review. While many similarities exist between secure development on Salesforce and a standard web application, there are also platform-specific vulnerabilities (and built-in security controls) to be aware of.
This guide is designed to help developers build secure Salesforce web applications, whether the goal is to pass the AppExchange review or to simply improve an application’s security posture. The seven sections below are organized by vulnerability class:
Each section provides a brief overview and two bulleted lists: one which explains how the vulnerability arises, the second detailing steps to remediate the vulnerability. By following these guidelines, your application will be more prepared to pass the Salesforce security review.
For more details on the security review process, check out the AppExchange Security Review Trailhead module.
CROSS-SITE SCRIPTING (XSS)
All standard VisualForce and Lightning components have built-in output encoding to prevent XSS from user-supplied data. XSS vulnerabilities typically arise when output is explicitly disabled (e.g. <span style="font-size:16px;background-color:#c2d6f2;">escape="false"</span>
). Avoid disabling the XSS protections offered by the platform, and if you must, use the HTMLENCODE or JSENCODE methods to manually apply output encoding. Also, keep in mind that several locations do not enforce automatic output encoding and should not include user-supplied input, as detailed below.
CAUSE OF XSS VULNERABILITIES |
<script>var userld = '{!$CurrentPage.parameters.username}';</script>
<style>body{background-color: {!$CurrentPage.parameters.bgColor};}</style>
<img src="image.jpg" onload="loadImage({!$CurrentPage.parameters.targetImage})">
<apex:includeScript value="{!$CurrentPage.parameters.scriptSrc}"/>
|
REMEDIATION OF XSS VULNERABILITIES |
|
References
Apex Developer Guide - Cross Site Scripting (XSS)
Secure Coding - Cross Site Scripting
Trailhead Units
Understand Cross-Site Scripting (XSS)
Discover Built-in XSS Protections in Lightning Platform
Prevent XSS in Lightning Platform Applications
CRUD/FLS
CRUD (Create, Read, Update, Delete) and FLS (Field Level Security) control user authorization and are important concepts on the SFDC platform. Salesforce data is represented as objects (e.g., Account, Case, and Lead. Object permissions for an organization are defined by the administrator account via profiles and permission sets. There are standard objects (built-in to the platform), and custom objects (which are created using Apex code). CRUD and FLS refer to different levels of authorization:
- CRUD settings enforce authorization at the object level - Account object
- FLS settings enforce authorization at the field level - Name, Phone Number, Website fields within the Account object
The SFDC platform can run in “user context” or “system context.”
User Context
When operating in user context, CRUD/FLS permissions are automatically enforced for the user’s current role. The platform executes in user context in these scenarios:
- When using the standard Salesforce UI with no custom Apex code
- When a VisualForce page uses a standard controller
- When a VisualForce page references objects using standard notation, also known as “referencing objects directly”:
<apex:pageBlockTable value="{!Account}" var="a"> <apex:column headervalue="Phone"> <apex:OutputText value="{!a.Phone}"/>
- When an application makes a standard API call (such as via the SFDC SOAP or REST APIs)
System Context
The SFDC platform supports system context to allow more flexible development, and is equivalent to running code as admin. Because system context does not automatically enforce authorization, enforcement must be added manually. The platform executes code in system context:
- When creating, reading, updating, or deleting a record with Lightning. Unlike VisualForce, Lightning does not support any built-in authorization.
- In custom Apex controllers or extensions to standard controllers
- In custom triggers
- In custom Apex web services
- When referencing objects indirectly from custom Apex code:
- Custom Controller:
public class productManager { public pagereference deleteProduct(){ productId = ApexPages.currentPage().getParameters().get('id'); Product__c delRecord = [SELECT ID FROM Product__c where id = :productId LIMIT 1]; delete delRecord
- VisualForce page:
<apex:page controller="productManager"> <apex:commandButton value="Delete" action="{!deleteProduct}">
- Custom Controller:
CAUSE OF CRUD/FLS VULNERABILITIES |
|
REMEDIATION OF CRUD/FLS VULNERABILITIES |
|
References
Enforcing CRUD and FLS
Trailhead Units
Learn How Authorization Works in Force.com Apps
Identify CRUD and FLS Violations in Visualforce and Apex
Prevent CRUD and FLS Violations
INSECURE SHARING
Sharing is similar to CRUD/FLS enforcement in that it controls authorization rights to a record. Whereas CRUD/FLS controls determine a user’s ability to access a specific object or field, sharing controls relate to a user’s ability to view, edit, or delete records belonging to other users. Imagine a class that includes a SOQL SELECT statement for several fields of an object:
String query = 'SELECT ClientName, Revenue from ConsultingAccounts__c';
If this class was declared without the with sharing
keyword, or with an explicit without sharing
, this query would return the records belonging to all users, not just the current user, even if the sharing settings for the object were configured as Private. Because Apex executes in system context, sharing enforcement must be explicitly declared to prevent users from accessing or modifying data that does not belong to them.
CAUSE OF INSECURE SHARING VULNERABILITIES |
|
REMEDIATION OF INSECURE SHARING VULNERABILITIES |
|
References
Using the with sharing, without sharing, and inherited sharing Keywords
Trailhead Units
Learn How Authorization Works in Force.com Apps
Identify and Prevent Sharing Violations
INSECURE STORAGE
It is common for Salesforce applications to store sensitive information including PII, credit cards, API keys, encryption keys, and passwords. Fortunately, the SFDC platform supports several methods for protecting sensitive data. The best option depends on the type of data being protected.
CAUSE OF INSECURE STORAGE VULNERABILITIES |
|
REMEDIATION OF INSECURE STORAGE VULNERABILITIES |
|
References
Storing Sensitive Data
Apex Developer Guide - Crypto Class
Trailhead Module
Secure Secret Storage
INSECURE EXTERNAL COMMUNICATION
Salesforce applications often integrate with third-party resources, either as part of a composite application or by simply including embedded resources or links from outside of Salesforce in a native application. This section details several best practices about integration with external resources.
CAUSE OF EXTERNAL COMMUNICATION VULNERABILITIES |
|
REMEDIATION OF EXTERNAL COMMUNICATION VULNERABILITIES |
|
References
Static Resources
Trailhead Unit
Use Static Resources
Prevent Insecure Remote Resource Interaction
SOQL INJECTION
Like regular SQL injection, SOQL (Salesforce Object Query Language) injection can be exploited to exfiltrate data from a database. The root cause is also the same as SQL injection - inserting user-controlled data directly into a SOQL query. In general, the best solution is also the same - binding user input to a variable and including that in a static query.
CAUSE OF SOQL INJECTION VULNERABILITIES |
|
REMEDIATION OF SOQL INJECTION VULNERABILITIES |
|
References
Apex Developer Guide - SOQL Injection
Trailhead Units
Understand SOQL Injection
Prevent SOQL Injection in Your Code
CROSS SITE REQUEST FORGERY
The SFDC platform automatically includes anti-CSRF tokens in forms created with VisualForce or Lightning (e.g., via <apex:form>
or <apex:commandLink>
). The only way to introduce this vulnerability is to perform a state-changing operation via GET
request, which occurs via the <apex:page action>
handler. By not doing this, the entire class of vulnerability can be avoided.
CAUSE OF CSRF VULNERABILITIES |
|
REMEDIATION OF CSRF VULNERABILITIES |
References
Apex Developer Guide - Cross-Site Request Forgery (CSRF)
Trailhead Unit
Prevent Cross-Site Request Forgery (CSRF)
Subscribe to our blog and advisories
Be first to learn about latest tools, advisories, and findings.
Thank You! You have been subscribed.